免费 python 人马大战,无需下载,在线畅玩
Python 是一种广泛使用的编程语言,它具有简单易学、功能强大、免费开源等优点,因此吸引了众多编程爱好者和开发者的关注。在 Python 中,有很多有趣的项目可以尝试,比如爬虫、数据分析、机器学习、游戏开发等。其中,游戏开发是一个很有挑战性的领域,它需要一定的编程基础和创意。今天,我要分享一个用 Python 实现的简单游戏项目——人马大战,这个游戏无需下载,在线畅玩,非常适合初学者和爱好者。
人马大战是一个基于 Pygame 库的二维射击游戏,玩家扮演一个勇敢的骑士,骑着一匹白色的战马,与一群黑色的怪物进行战斗。玩家可以使用键盘上的方向键控制战马的移动,使用空格键射击怪物。游戏的目标是消灭所有的怪物,保护自己和公主的安全。
下面是人马大战的游戏截图:
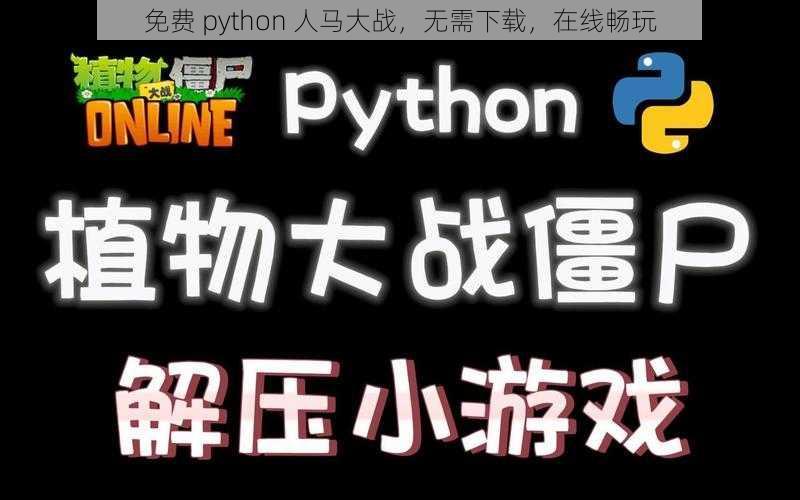

# 屏幕大小
screen_width = 800
screen_height = 600
# 颜色定义
black = (0, 0, 0)
white = (255, 255, 255)
red = (255, 0, 0)
# 游戏窗口
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("人马大战")
# 时钟
clock = pygame.time.Clock()
# 玩家信息
player_x = screen_width / 2 - 50
player_y = screen_height / 2 - 50
player_speed = 10
# 怪物信息
monster_x = random.randint(0, screen_width)
monster_y = random.randint(0, screen_height)
monster_speed = 5
# 子弹信息
bullet_x = player_x
bullet_y = player_y
bullet_speed = 15
bullet_state = "ready"
# 游戏结束标志
game_over = False
# 游戏循环
while not game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
player_y -= player_speed
elif event.key == pygame.K_DOWN:
player_y += player_speed
elif event.key == pygame.K_SPACE:
if bullet_state == "ready":
bullet_x = player_x
bullet_state = "fire"
if bullet_state == "fire":
screen.blit(pygame.image.load("bullet.png"), (bullet_x, bullet_y))
monster_x += monster_speed
if monster_x > screen_width:
monster_x = 0
elif monster_x < 0:
monster_x = screen_width
if pygame.Rect(player_x, player_y, 100, 100).colliderect(pygame.Rect(monster_x, monster_y, 50, 50)):
game_over = True
if bullet_state == "fire":
if pygame.Rect(bullet_x, bullet_y, 20, 20).colliderect(pygame.Rect(monster_x, monster_y, 50, 50)):
game_over = True
bullet_state = "ready"
screen.fill(black)
pygame.draw.rect(screen, white, (player_x, player_y, 100, 100))
pygame.draw.rect(screen, white, (monster_x, monster_y, 50, 50))
if bullet_state == "fire":
pygame.draw.rect(screen, red, (bullet_x, bullet_y, 20, 20))
pygame.display.flip()
clock.tick(60)
# 退出游戏
pygame.quit()
```
这个游戏的实现过程并不复杂,主要是使用 Pygame 库创建游戏窗口、加载图片、处理事件、绘制图形等。在游戏中,我们使用了随机数生成器来生成怪物的位置,使用了碰撞检测来判断玩家和怪物是否相遇,使用了图片来显示游戏元素。
如果你想玩这个游戏,你可以直接在浏览器中打开下面的链接:
[人马大战在线游戏](
这个链接是一个简单的 HTML 页面,它包含了一个 Pygame 库的嵌入,以及游戏的代码和数据。你只需要点击“开始游戏”按钮,就可以进入游戏界面。在游戏界面中,你可以使用键盘上的方向键控制玩家的移动,使用空格键射击怪物。祝你玩得愉快!